Using the JSON API
Integrates your bot with API. Gives an opportunity to generate and post custom content in a chat.
To illustrate how you can generate and post custom content via API, here is a demonstration of how you can create a Weather Forecast Chatbot that provides a weather forecast for a selected location and period using the API of the weather forecast web service (here provided by SnatchBot).
When creating a bot, there is the option of selecting a template that is already configured and ready for communication with the customer. In this case, import the WeatherBot.
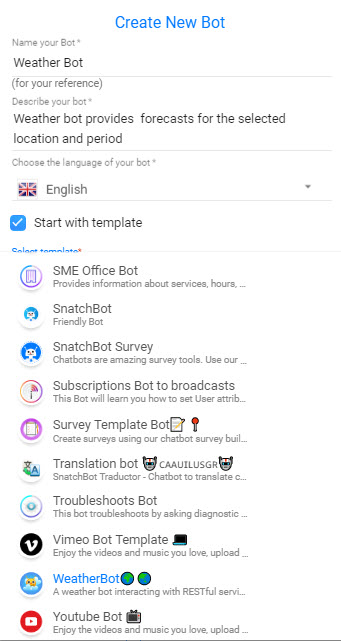
Depending on the customer’s answers, the bot sends API requests to the web service to get the responses that will be processed and shown to the customer as cards (.png format).
To start with, the bot should understand how the customer would like to get a weather forecast: by city or by postcode (type). For example, the customer might choose, ‘by city’. To connect the request to the web service, we need to know the name of the city, for instance, Madrid.
Next, using the JSON API interaction in the bot’s structure, SnatchBot sends information about the customer’s city to the third-party server (weather.snatchbot.me
) - https://weather.snatchbot.me/?type=setCity
- where ‘type=setCity’ is a query parameter to save the city in the third-party database.
Along with this parameter, SnatchBot server sends a request via POST method (bot_id, user_id, module_id, channel, incoming_message parameters). After that, the third-party server processes the request, saves type, user_id and customer’s city to the database and the third-party server sends JSON response to the Snatchbot’s server.
Here is an example of how third-party JSON response is obtained and used (the program is written on PHP in PhpStorm development environment):
// Data sent via POST method is saved to the appropriate variables
$bot_id = $_POST['bot_id'];
$user_id = $_POST['user_id'];
$module_id = $_POST['module_id'];
$channel = $_POST['channel'];
// Add a message that will be displayed in the chat
$message = 'I can tell you what the weather is like today!';
// Add quick replies to the chat
$suggestedReplies = ["Today's weather",'Next 5 days','Pick a day', 'Return to the beginning'];
// Add answer’s header
header ('Content-Type: application/json');
// Build an array $response
$response = [
'user_id' => $user_id,
'bot_id' => $bot_id,
'module_id' => $module_id,
'message' => $message,
'suggested_replies' => $suggestedReplies,
'blocked_input' => false
];
// Convert an array to JSON string and send as a server response to the Snatchbot server
echo json_encode($response);
// End program
die();
For the customer’s convenience in the previous step, we’ve added quick replies ($suggestedReplies). For instance, customer selected ‘Today’s weather’.
Next, we need to create a JSON API Interaction with the following string in the API address field – https://weather.snatchbot.me/?type=oneDayCity
(where wheather.snatchbot.com is API address of the third-party server and ‘type=oneDayCity’ is a query parameter to show current weather in the selected previously city).
The SnatchBot server will send a request to the third-party server; it will save a city (for user_id) in its database, send a request to the OpenWeather server, and, after it receives a response from the OpenWeather server, processes it, and return a JSON response to the Snatchbot’s server.
Here is an example (including the option to convert HTML to .png to display for the customer in the chat):
//Using Composer install Html2Pdf library
//Import library
require_once '/vendor/autoload.php';
use Spipu\Html2Pdf\Html2Pdf;
use Spipu\Html2Pdf\Exception\Html2PdfException;
use Spipu\Html2Pdf\Exception\ExceptionFormatter;
if $_GET["type"] == "oneDayCity" {
// Call oneDayCity() function to make a request to OpenWeatherMap API and receive JSON response
$jsonOpenweather = oneDayCity();
// Convert JSON to an array
$arrayOpenweather = json_decode(jsonOpenweather);
// Data sent via POST method is saved to the appropriate variables
$bot_id = $_POST['bot_id'];
$user_id = $_POST['user_id'];
$module_id = $_POST['module_id'];
$channel = $_POST['channel'];
// Add a message that will be displayed in the chat
$message = 'Here u are!'\n;
$message += 'The temperature is'+$arrayOpenweather["main"]["temp"];
// Add quick replies to the chat
$suggestedReplies = ['Next 5 days','Pick a day', 'Return to the beginning'];
// Add answer’s header
header('Content-Type: application/json');
// Build an array $response
$response = [
'user_id' => $user_id,
'bot_id' => $bot_id,
'module_id' => $module_id,
'message' => $message,
'suggested_replies' => $suggestedReplies,
'blocked_input' => false
];
// Convert an array to JSON string and send as a server response to the Snatchbot server
echo json_encode($response);
// End program
die();
}
function oneDayCity() {
$options = array(
CURLOPT_RETURNTRANSFER => true, // return web page
CURLOPT_HEADER => false, // don't return headers
CURLOPT_FOLLOWLOCATION => true, // follow redirects
CURLOPT_MAXREDIRS => 10, // stop after 10 redirects
CURLOPT_ENCODING => "", // handle compressed
CURLOPT_USERAGENT => "test", // name of client
CURLOPT_AUTOREFERER => true, // set referrer on redirect
CURLOPT_CONNECTTIMEOUT => 120, // time-out on connect
CURLOPT_TIMEOUT => 120, // time-out on response
);
$ch = curl_init("http://api.openweathermap.org/data/2.5/weather?q=London&units=metric&APPID=3dd56b9c2b2dc702d326f13bc0859ca7");
curl_setopt_array($ch, $options);
$content = curl_exec($ch);
curl_close($ch);
return $content;
}
function oneDayCity($data) {
$html = '<style>
.outer-div {
position: relative;
width: 100%;
height: 100%;
background-color: #E6F4FF;
text-align: center;
font-weight: bold;
letter-spacing: 0.4px;
}
.outer-div p {
margin: 0;
}
.top-div {
position: absolute;
top: 4%;
left: 5%;
width: 90%;
height: 10%;
padding: 20px 0;
background-color: #0388C0;
color: #ffffff;
}
.top-div .city {
font-size: 45px;
}
.top-div .date {
padding-top: 10px;
font-size: 30px;
}
.bottom-div {
position: absolute;
top: 175px;
left: 5%;
width: 90%;
height: 80%;
background-color: #ffffff;
color: #0388C0;
padding-bottom: 15px;
}
.bottom-div .img-w{
width: 250px;
height: 250px;
}
.bottom-div .t{
font-size: 125px;
margin-top: 15px;
}
.bottom-div .max-t,
.bottom-div .min-t{
font-size: 67px;
}
.bottom-div .max-t{
margin-top: 40px;
margin-bottom: 5px;
}
.bottom-div .min-t{
margin-top: 5px;
margin-bottom: 40px;
}
.bottom-div .cloud,
.bottom-div .wind,
.bottom-div .pressure,
.bottom-div .humidity{
font-size: 40px;
margin: 10px auto;
}
</style>';
$html += '<div class="outer-div">
<div class="top-div">
<p class="city">' + strtoupper($data->name) . ', ' . $data->sys->country + '</p>
<p class="date">' + date('l', $data->dt) . ', ' . strtoupper(date('M', $data->dt)) . ' ' . date('d', $data->dt) + '</p>
</div>
<div class="bottom-div">
<img class="img-w" src="' + dirname(__FILE__) . '/../images/wapi/'. $data->weather['0']->icon + '.png">
<p class="t">' + round($data->main->temp) + '°C</p>
<p class="max-t">Max: ' + $data->main->temp_max + '°C</p>
<p class="min-t">Min: ' + $data->main->temp_min + '°C</p>
<p class="cloud">' + $data->clouds->all + '% Cloud cover</p>
<p class="wind">' + $data->wind->speed + ' m/s Wind speed</p>
<p class="pressure">' + $data->main->pressure + ' mm Hg Pressure</p>
<p class="humidity">' + $data->main->humidity + '% Humidity</p>
</div>
</div>';
return $html;
}
function getImage() {
$img_name = time();
try {
ob_start();
// Generate and save HTML
$content = oneDayCity($jsonOpenweather)
$html2pdf = new Html2Pdf($rotate, $size, 'en', true, 'UTF-8', array(0, 0, 0, 0));
$html2pdf->writeHTML($content);
$html2pdf->pdf->SetDisplayMode('fullwidth');
$html2pdf->setDefaultFont('OpenSans');
$html2pdf->output('/pdf/' . $img_name . '.pdf', 'F');
} catch (Html2PdfException $e) {
$formatter = new ExceptionFormatter($e);
echo $formatter->getHtmlMessage();
}
$im = new imagick(dirname(__FILE__).'/pdf/' . $img_name .'.pdf');
$d = $im->getImageGeometry();
$w = $d['width'];
$h = $d['height'];
if ($w==false) {
$width = $w;
}
$im->setImageFormat( "png" );
$im->setImageBackgroundColor('white');
$im->resizeImage( $width, $height, Imagick::FILTER_LANCZOS, 1);
$im->setCompressionQuality(100);
$img_name_path = dirname(__FILE__).'/images/' . $img_name .'.png';
$im->writeImage($img_name_path);
$im->clear();
$im->destroy();
$root = 'http://95075b72.ngrok.io';
$img_url = $root . '/images/' . $img_name .'.png';
return $img_url
}
For the following API request to the OpenWeather server: http://api.openweathermap.org/data/2.5/weather?q=London&units=metric&APPID=3dd56b9c2b2dc702d326f13bc0859ca7 the JSON response below will be returned:
{
"coord":
{
"lon":-0.13,
"lat":51.51
},
"weather":
[
{
"id":721,
"main":"Haze",
"description":"haze",
"icon":"50d"
},
{
"id":701,
"main":"Mist",
"description":"mist",
"icon":"50d"
},
{
"id":301,
"main":"Drizzle",
"description":"drizzle",
"icon":"09d"
}
],
"base":"stations",
"main":
{
"temp":9.69,
"pressure":1007,
"humidity":81,
"temp_min":8,
"temp_max":11
},
"visibility":5000,
"wind":
{
"speed":2.1,
"deg":30
},
"clouds":
{
"all":90
},
"dt":1523460000,
"sys":
{
"type":1,
"id":5091,
"message":0.0028,
"country":"GB",
"sunrise":1523423543,
"sunset":1523472689
},
"id":2643743,
"name":"London",
"cod":200
}
After that, a response is sent to the third-party server where it is processed, converted and sent to the Snatchbot’s server as an answer to the customer (cards in the .png format will be displayed in the chat):
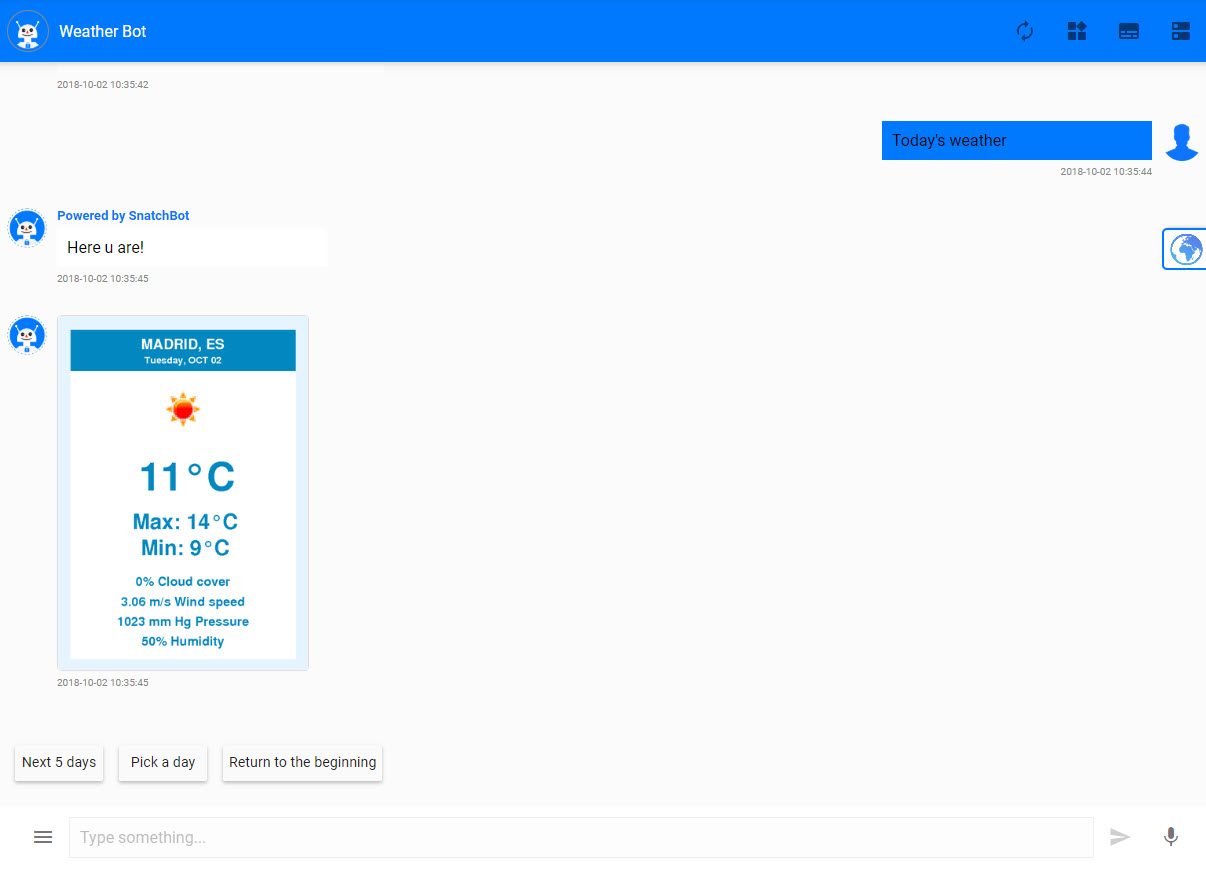
Watch this video to learn more about how to use the JSON API with SnatchBot
Updated over 3 years ago
How to Connect Your Chatbot With Third-Party APIs With the Help of Integromat